binary tree python insert
Def insert root node. The Python code for implementation of Binary Search Tree is given below.
A tree is an instance of a more general data structure called a graph.
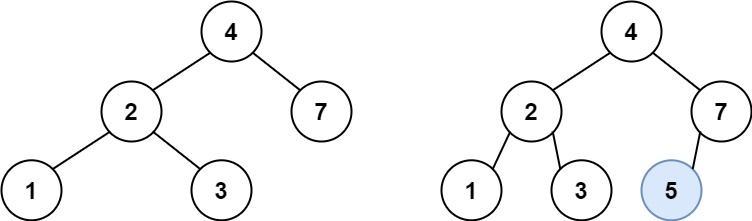
. If data selfdata. Insertion in a Binary Tree in level order. Create a function to insert the given node and pass two arguments to it the root node and the data to be inserted.
Selfleft None selfright None selfdata data def insertself data. Selfdata data def getTreeself. Selfleft None selfright None selfvalue value def insertself value.
Related
If binary search tree is empty make a new node and declare it as root if root is None. Def _init_ self key Put and initialize the key and value pait. Given a binary tree and a key insert the key into the binary tree at the first position available in level order.
If nodeleft is None. Return The Binary Heap is fully occupied. Compare the inserting element with root if less than root then recursively call left subtree else recursively call right subtree.
This becomes tree with only a root node. The inversion of a binary tree or the invert of a binary tree means to convert the tree into its mirror image. Nodevalue value nodeleft None noderight None def Inorder node Root.
Right None self. Selfvalue value def PrintTreeself. Following the code snippet each image shows the execution visualization which makes it easier to visualize how this code works.
Right key return the unchanged node pointer. After reaching the end just insert that node at leftif less than current else right. To implement a binary tree in python you should know the basic python syntax.
If selfleft is None. Insertion in Binary search tree BST in Python class Tree. If selfleft is None.
Selfleft Treedata else. If selfright is None. Return Node key Otherwise recur down the tree.
Given the root of a binary tree invert the tree and return its root. If rootl_child is None. Def __init__self data left None right None.
Selfleft BinaryTreevalue else. If root is None. Insert rootl_child node else.
Function to Insert a Node in Binary Heap def inserNoderoot_node nodeValue heapType. Value key A function to insert a new node with the given key value def insert rootnode. Define a temporary node to store the popped out nodes from the queue for search purpose.
Python - Binary Tree Create Root. RootBinaryTreeNodenewValue return root binary search tree is not empty so we will insert it into the tree if newValue is less than value of data in. Selfright BinaryTreevalue else.
If root is None. Selfleftinsertvalue elif data selfvalue. The syntax flow for the binary search Tree in Python is as follows.
We just create a Node class and add assign a value to the node. Selfleftinsertdata elif data selfdata. Binary Tree in Python.
If root is None. Return node Given a non-empty binary search tree return the node with minum key value found in. Return 0 else.
Compute the height of left and right subtree l_height heightrootleft r_height. If treeright None. If treeleft None.
If node is None. This is a simple implementation of Binary Search Tree Insertion using Python. Left insert node.
If data selfvalue. Inserting into a Tree. Return nodeInorderRootleft printRootvalueend nodeInorderRootright def Insertnode value.
Python program to demonstrate insert operation in binary search tree class Node. Rootl_child node else. 36 rows insertrootleft node code.
Def insert node key. If node is None. See what employees say it s like to work at geeksforgeeks.
Selfdata data self View the full answer. Define a queue data structure to store the nodes of the binary tree. Root node else.
If selfright is None. To insert into a tree we use the same node class created above and add a insert class to. Then a function call to heapifyTreeInsert is made to adjust the newly inserted to its rightful position in the binary heap.
Constructor with vaue we are going to insert in tree with assigning left and right child with default None def __init__self data. Selfright Treedata else. If key node.
Listing 2 shows the Python code for a preorder traversal of a binary tree. InsertTree element return Tree def heightroot. Level Order traversal of the binary tree is.
Root node else. 1 2 3 output. Left None self.
Node Treevalue elif value nodevalue. Then a utility function as per requirement can be written. Treeleft Treeitem else.
To implement a binary search tree we will use the same node structure as that of. If rootdata nodedata. If the tree is empty return a new node.
If binary search tree is empty make a new node and declare it as root if root is None. 50 20 53 11 22 52 78 In the above program we have first implemented the binary search tree given in the figure. To insert into a tree we use the same node class created above and add a insert class to.
Start from the root. Selfdata value selfleft l. Binary Tree in Python class Node.
Illustration to insert 2 in below tree. If Root is None. Binary tree python geeksforgeeksThe time complexity of binary search can be written as.
Tree TreeNodeelements0 for element in elements1. If item treeentry. Def insertitem tree.
Finally the PrintTree method is used to print the tree. Selfdata data selfleft left selfright right def make_treeelements. Right insert node.
If root_nodeheapSize 1 root_nodemaxSize. There are three ways of traversing a binary tree. Program to invert a binary tree in python.
Selfdata data selfleftChild None selfrightChildNone def insertrootnewValue. Def insert root key define function. To add an element into a binary tree we just need to write the insert function compares the value of the node to the parent node and decides to add it as a left node or a right node.
Insert Into A Binary Search Tree Leetcode
Binary Search Trees Bst With Code In C Python And Java Algorithm Tutor
Level Order Traversal Of Binary Tree Python Code Favtutor
Build The Forest In Python Series Binary Tree Traversal Shun S Vineyard
Deletion In A Binary Tree Geeksforgeeks
Binary Search Tree Implementation 101 Computing
Insertion In A Binary Tree In Level Order Geeksforgeeks
Binary Search Tree Complete Implementation In Java Algorithms Binary Tree Data Structures Machine Learning Deep Learning
Check Whether The Leaf Traversal Of Given Binary Trees Is The Same Or Not Techie Delight
Print Complete Binary Search Tree Bst In Increasing Order Techie Delight
Ways To Traverse A Binary Tree Using The Recursive And Iterative Approach By Wangyy Medium
Height Of A Binary Tree Python Code With Example Favtutor
Binary Tree Methods In Python Kevin Vecmanis
Binary Tree Binary Tree Binary Doodles
Insert Into A Binary Search Tree Leetcode
Difference Between Binary Tree And Binary Search Tree Geeksforgeeks
Binary Search Tree Using Python
Python Program To Implement Binary Search Tree Program 3 Search Operation Python Programming Binary Tree Data Structures
Check If Removing An Edge Can Split A Binary Tree Into Two Equal Size Trees Techie Delight